CUCM AXL API – Removing Directory Numbers Using Python
In this blog, we are going to demonstrate how we can remove or delete multiple directory numbers from CUCM using CUCM AXL API using Python. We will be using SOAP UI and Postman to demonstrate the same and then will use Python to implement it.
Setting Up AXL API User for CUCM
Open CUCM administration page and navigate to “User Management” and then select Application User
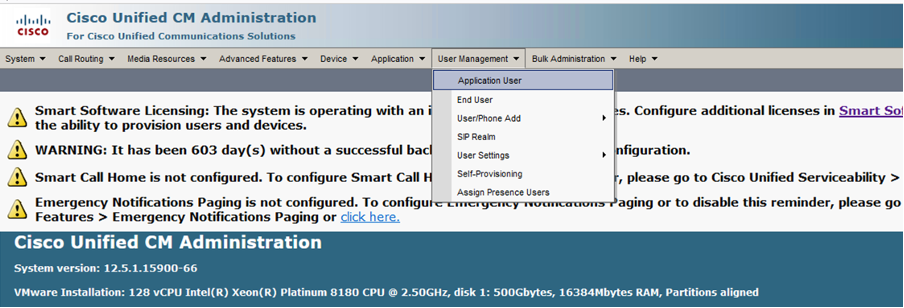
Then navigate to “User Settings” and select “Access Control Group”
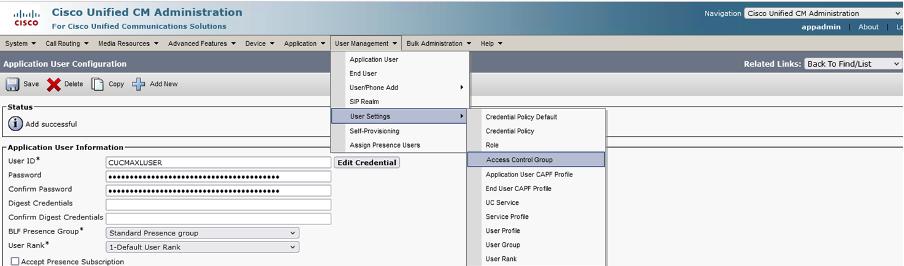
Click on Add New and give a name to your access control group and then select “Assign Role to Access Control Group” from the drop-down list located on the right top corner
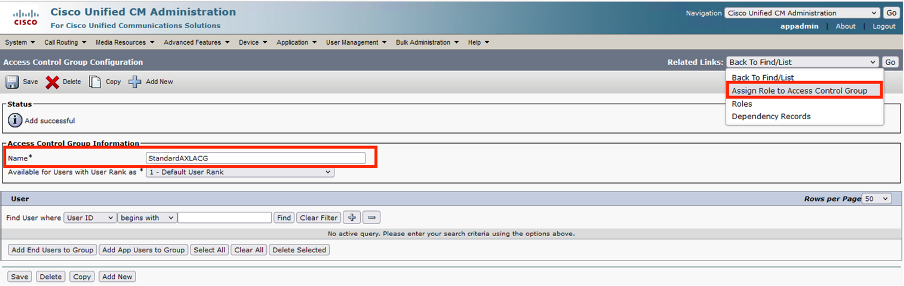
Select Standard AXL API Access and click on add selected. Now you have assigned the required role to your ACG. The next task is to assign this ACG to your new application user.
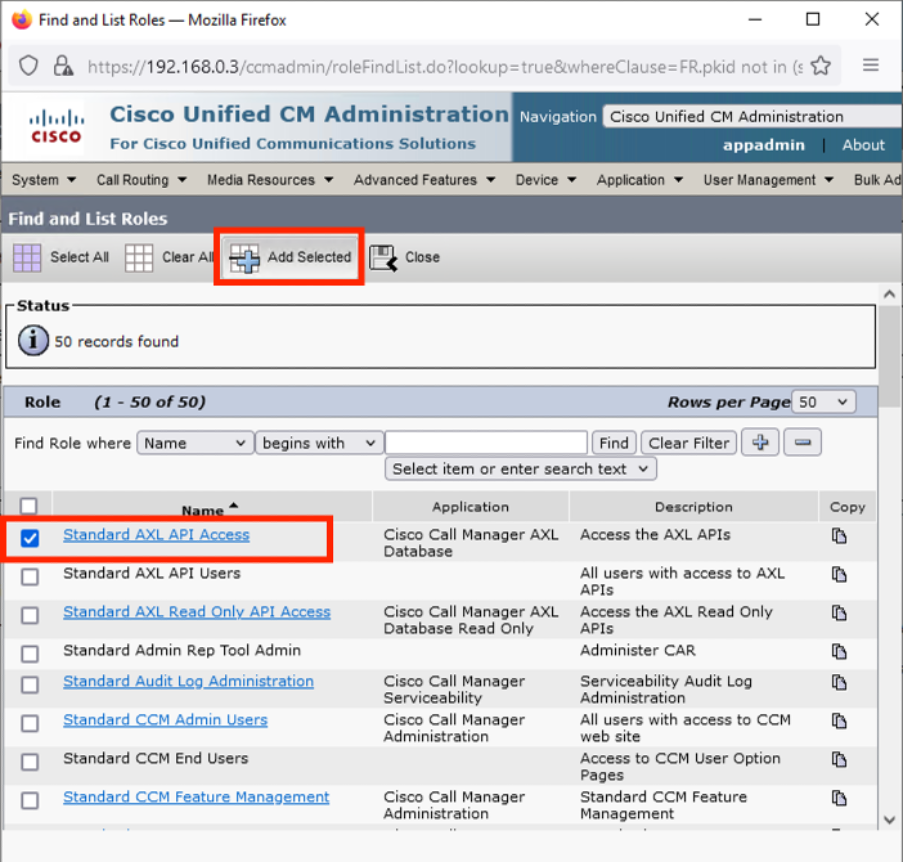
Navigate to the bottom of the application user and then select “Add to access Control Group” and then select the ACG that we have created.
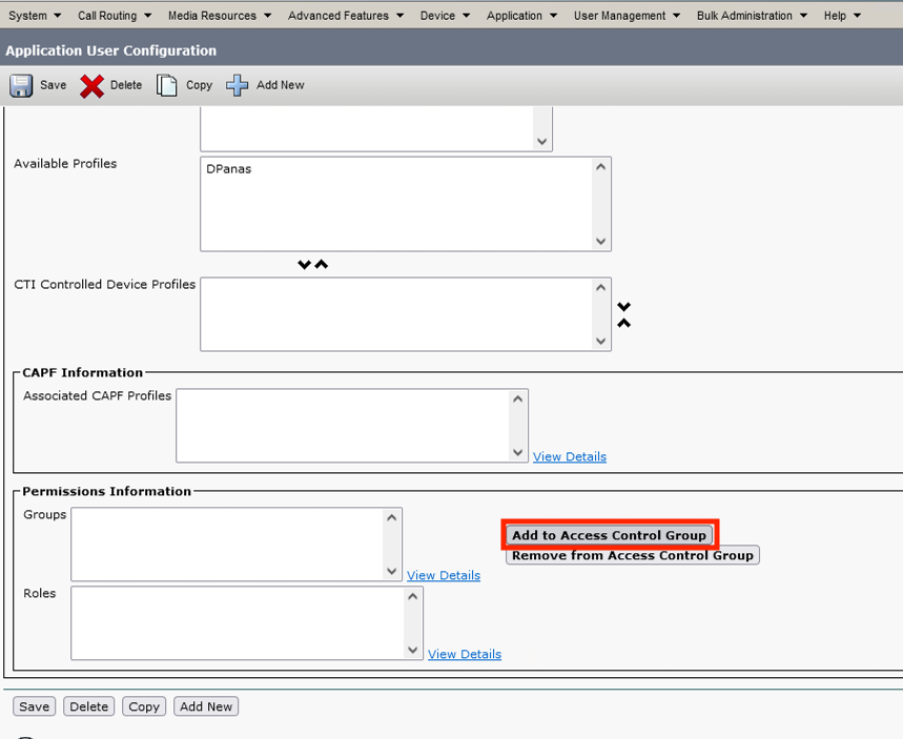
It will list all the ACGs available and then select the one we created for API access and hit save. Once saved, the roles section will list like below
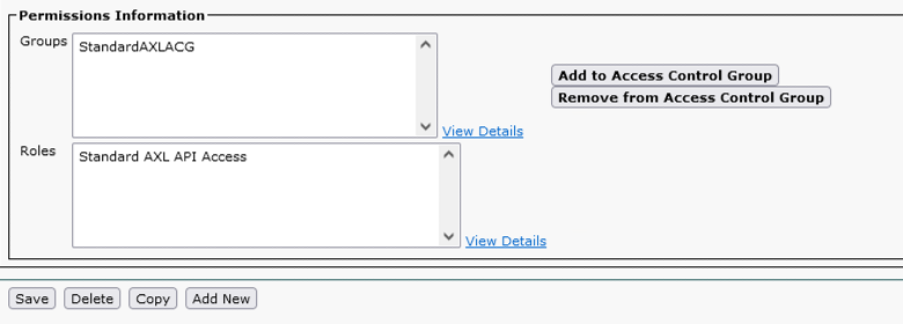
Downloading Cisco AXL Toolkit from CUCM
The first step to set up the CUCM AXL automation environment is to have the CISCO AXL tool kit downloaded. It can be done by navigating to the administration page of the CUCM and then go to the Application dropdown menu and select plugins, inside the plugins section, you will find “Cisco Axl Toolkit”, download it, downloaded file a zip file name “axlsqltoolkit.zip”.
Extract the zip file and make a note of the directory, we are going to point that to the SOAP UI application in a while
Inside this downloaded file we have the WSDL file which defines the structure and interface of the AXL web service, making it easier for developers to generate test code and communicate with CUCM through API calls. WSDL files provide a standardized way to describe web services, including the operations they support, the data types they use, and the endpoints where they can be accessed.
Download the SOAP UI Application
Download the SOAP UI application from the below link and then install it by default settings
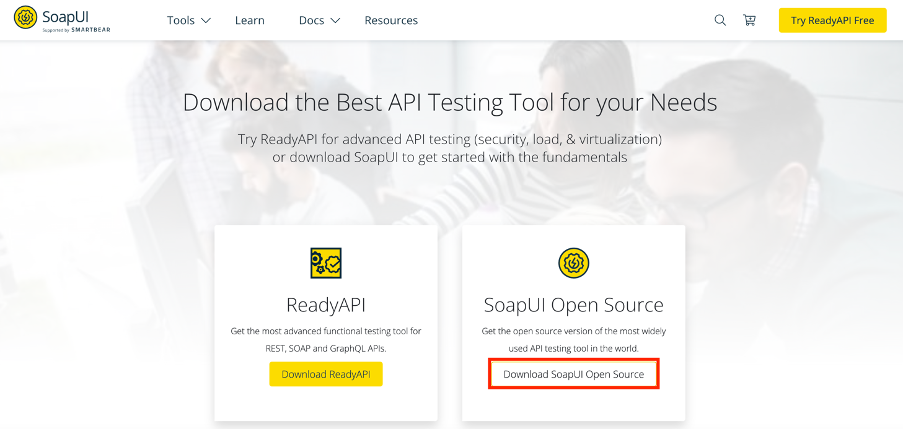
Linking the WSDL file to the SOAP UI application
Open the SOAP UI application, where you may notice several default windows opening to assist you in navigating through its features, similar to what you might encounter when launching any other application for the first time. Close those windows and return to the home or default view of the SOAP UI application and click on “New SOAP Project”

It opens a window where you can feed the project name of your choice and point the WSDL file to it
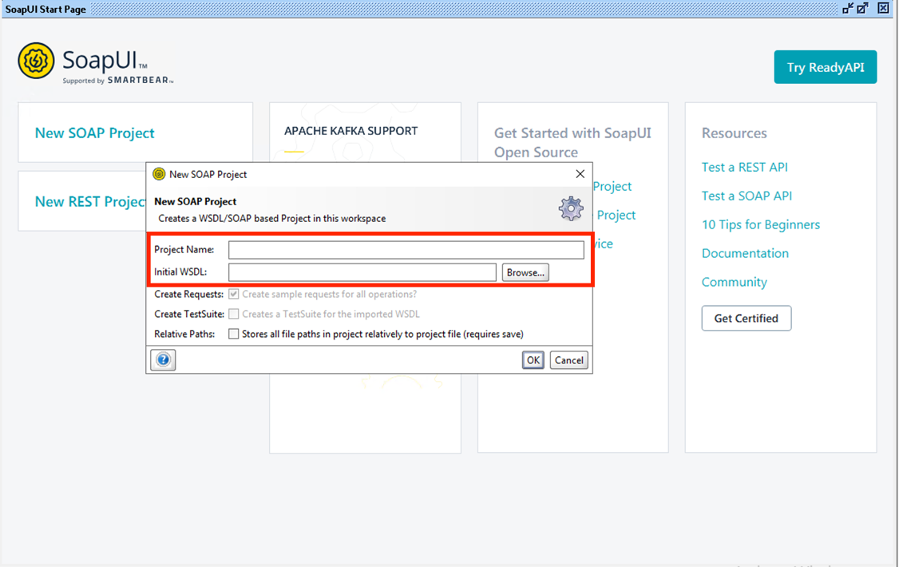
Click on browse and navigate to the directory where you downloaded and placed the Cisco AXL toolkit, open the directory and then open the schema folder, it lists a couple of directories with CUCM versions, open the appropriate directory and then select the file with the WSDL extension, usually the file is named as AXLAPI.wsdl.
After this, your project name will be listed under the Projects section below
Connecting SOAP UI to Cisco CUCM
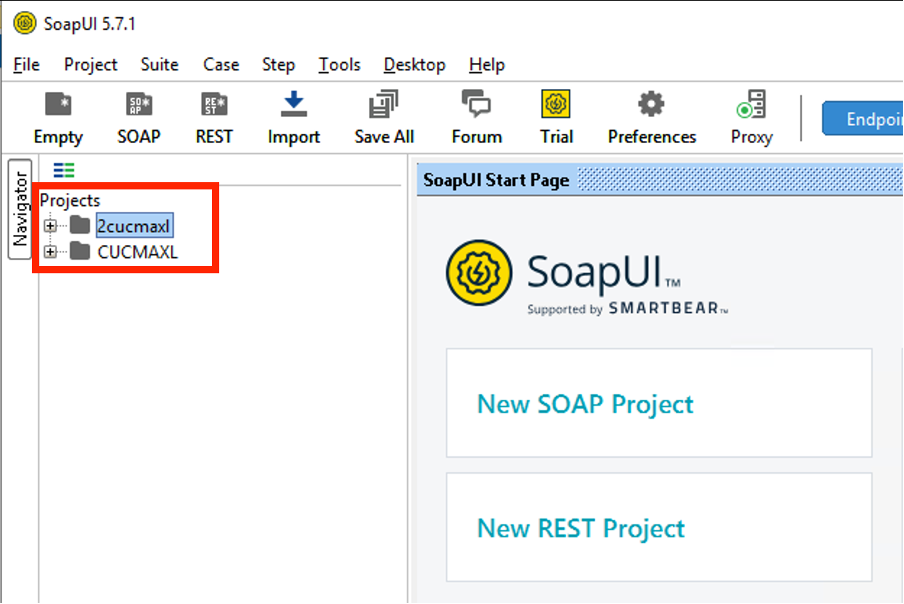
Expand your project directory and then select the first option which is AXLAPIBinding and do double click on it or right-click and then open “show interface viewer”
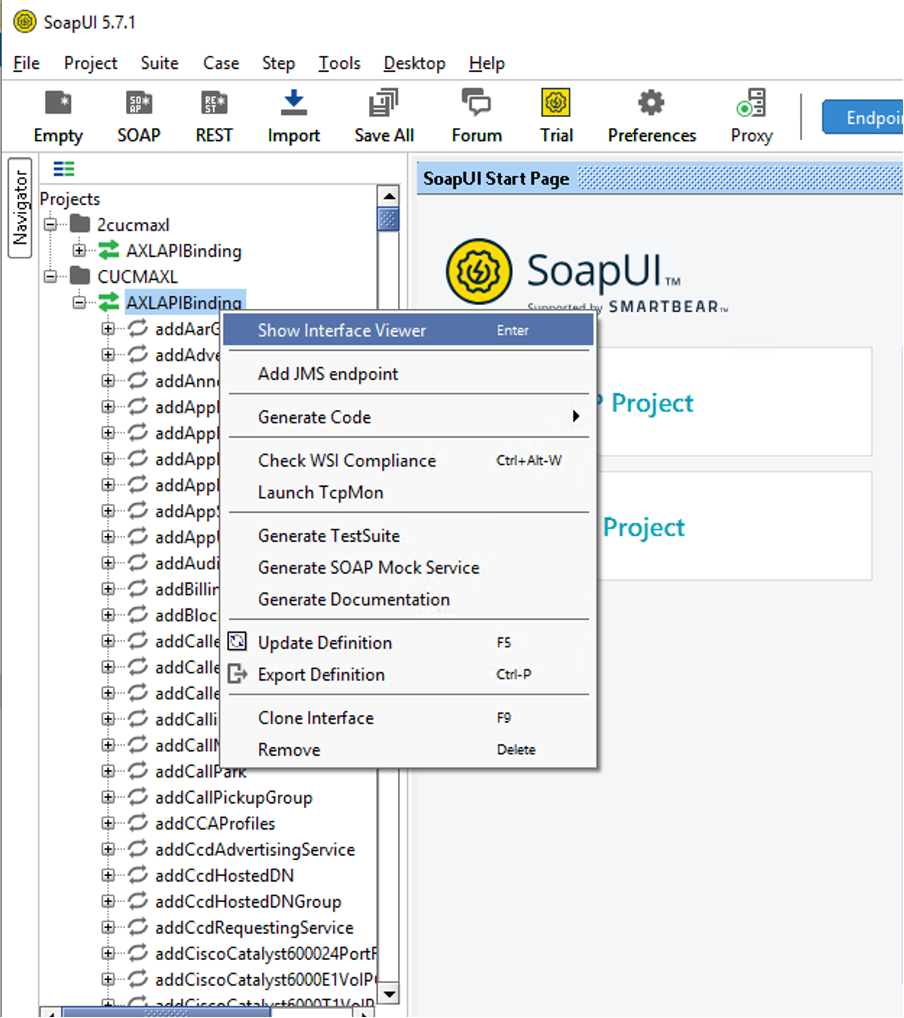
Then replace the CCMSERVERNAME with your CUCM IP address or hostname in the endpoint field and then provide the AXL username in the username field and password in the password field.
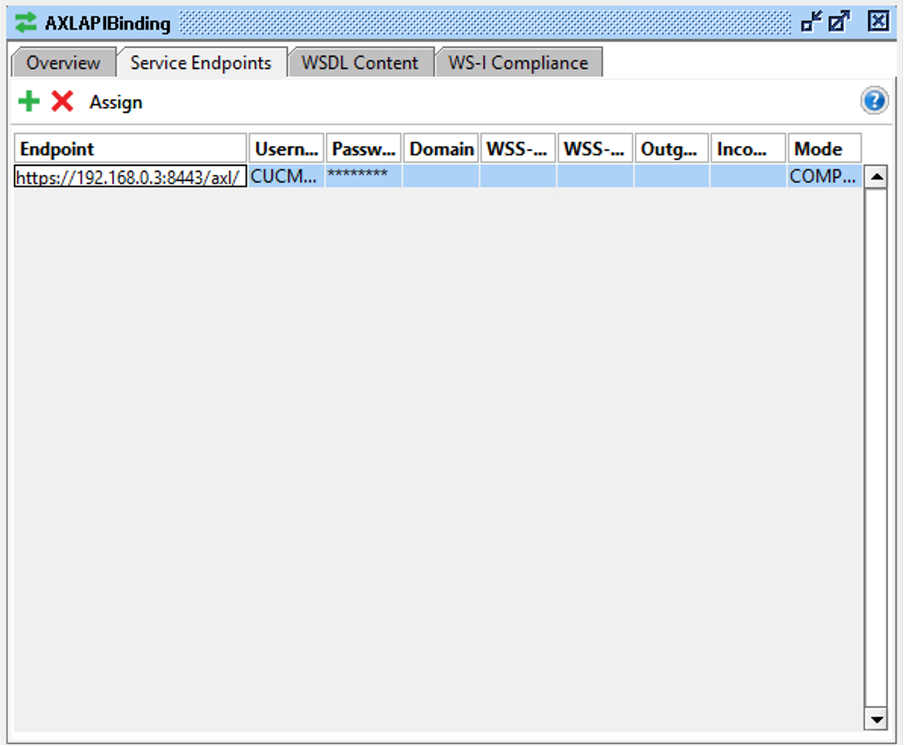
Close the AXLAPIBinding window and now are done with the configuration.
Testing the setup by sending CCMGetVersion Command
The next task is to test its working of, for we will use the simplest command CCMGetversion to display the version of the system.
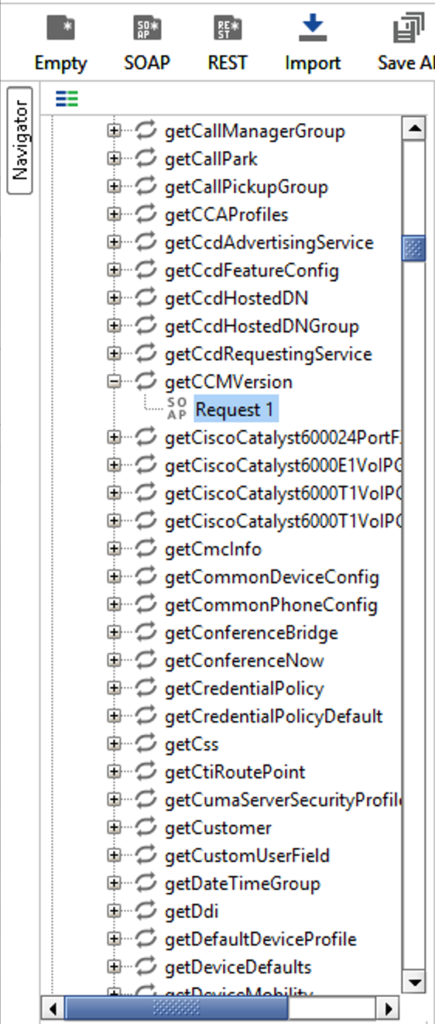
Navigate to the getCCMVersion command and then expand it to get the request command
Double-click on the command
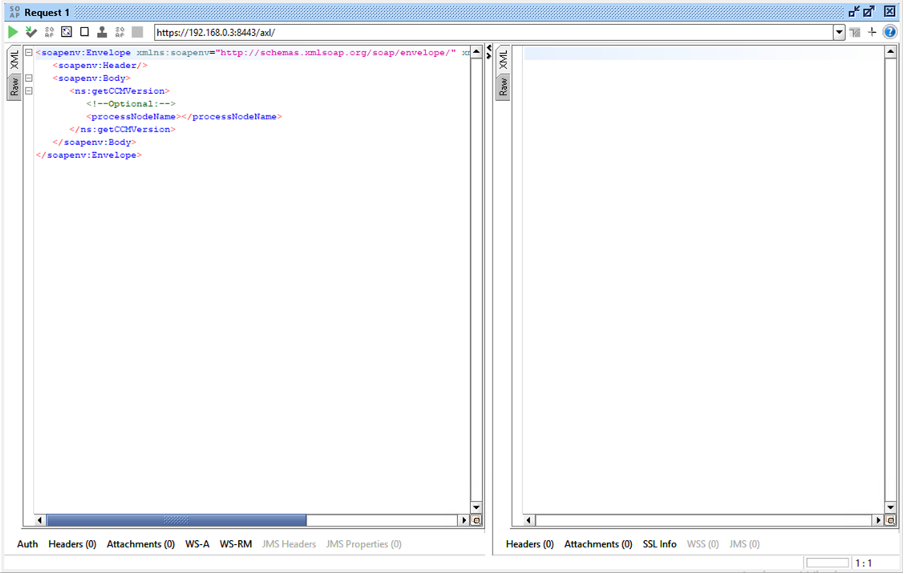
You will see the XML format request, remove the question mark in the processnodename XL element
<processNodeName></processNodeName>
And then submit the request by hitting the green play button.
The result will displayed on the right pane section where you have the option to select raw output data or the XML content, here I have displayed the XML content.
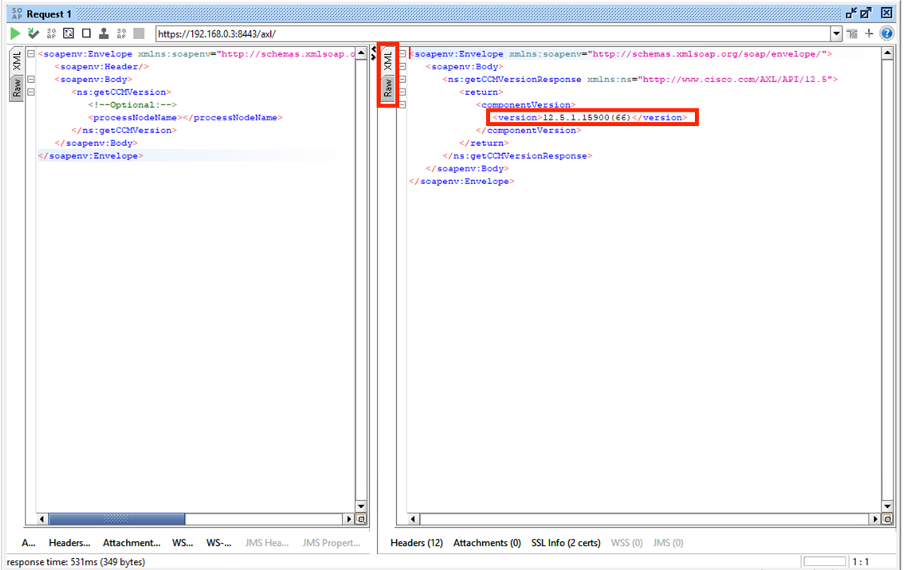
Going for Remove Line Command
At this point, we have verified our request is working and now we can implement the Python code to delete multiple directory numbers by supplying it from an Excel sheet.
Like I have selected getccmversion command, here I have selected remove line command
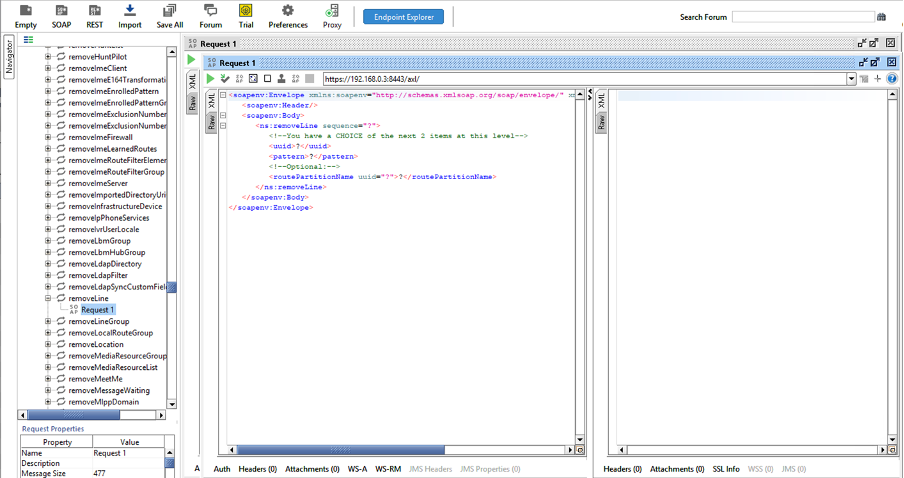
I am removing the ‘uuid’ element as I am giving the directory number and ‘routePartitionName’ name to uniquely identify the name pattern, and then I have to remove the question mark from the ‘pattern’ element, supplying the directory number of my interest. Additionally, I must provide the ‘routePartitionName’ value by removing the question mark there.
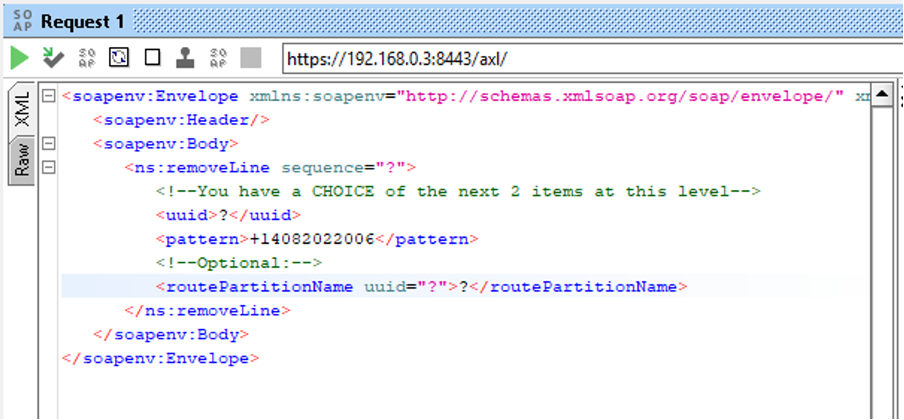
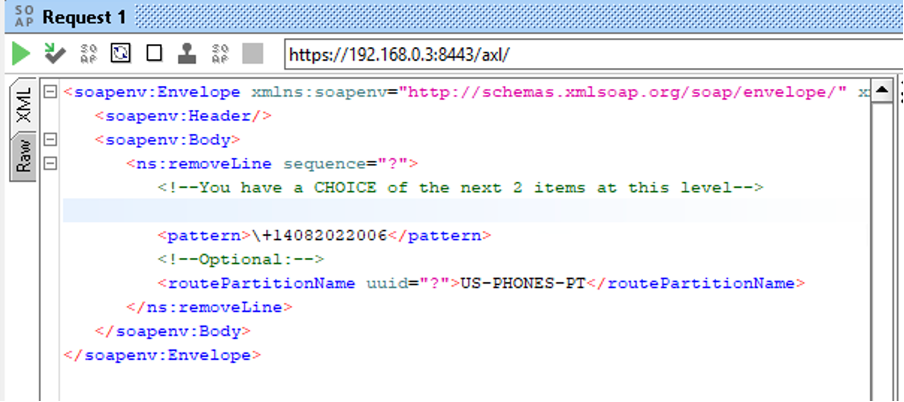
Below is how my directory number is listed in the CUCM Directory Number Section under Call Routing.
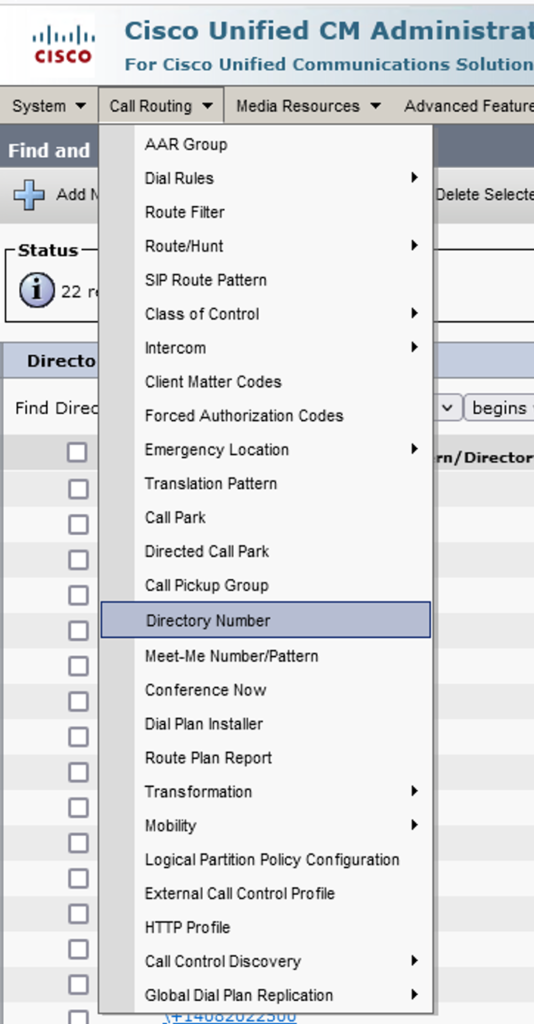
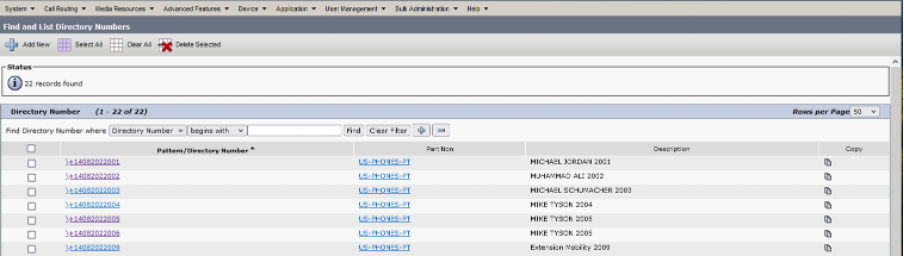
Now I am defining a Python function to define the SOAP request payload
Python Code
import requests
import base64
import pandas as pd
# Define the AXL API URL
url = "https://hq-cucm-pub.ciscouc.com/axl/"
# Define the path to the Excel file
excel_file_path = "data.xlsx"
# Read data from the Excel file using pandas
data = pd.read_excel(excel_file_path)
# Define username and password
username = "axlapiuser"
password = "axlapiuser"
# Define request headers, including Basic Authentication
headers = {
'Content-Type': 'text/xml',
'Authorization': f'Basic {base64.b64encode(f"{username}:{password}".encode()).decode()}'
}
def send_remove_line_request(pattern, route_partition_name):
# Define the SOAP request payload
payload = f"""
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:ns="http://www.cisco.com/AXL/API/12.5">
<soapenv:Header/>
<soapenv:Body>
<ns:removeLine sequence="?">
<pattern>{pattern}</pattern>
<routePartitionName uuid="?">{route_partition_name}</routePartitionName>
</ns:removeLine>
</soapenv:Body>
</soapenv:Envelope>
"""
try:
# Send a POST request to the AXL API
response = requests.post(url, headers=headers, data=payload, verify=False)
# Check if the request was successful (status code 200)
if response.status_code == 200:
print(f"Request for pattern '{pattern}' and routePartitionName '{route_partition_name}' was successful. Response:")
print(response.text)
else:
print(f"Request for pattern '{pattern}' and routePartitionName '{route_partition_name}' failed with status code: {response.status_code}")
print(response.text)
except requests.exceptions.RequestException as e:
print(f"An error occurred while sending the request: {e}")
if __name__ == "__main__":
# Loop through the rows of data and send SOAP requests for each combination
for index, row in data.iterrows():
pattern = row["pattern"]
route_partition_name = row["routePartitionName"]
send_remove_line_request(pattern, route_partition_name)
Github Link: https://github.com/anashira/CUCM-AXL-API.git
Copy the XML content from the SOAP UI application and place it in the payload variable, also rename the actual value with variables In this case it is pattern and routePartitionName
Below is the screenshot of my sample Excel where I supplied the directory number and RoutePartitionName.
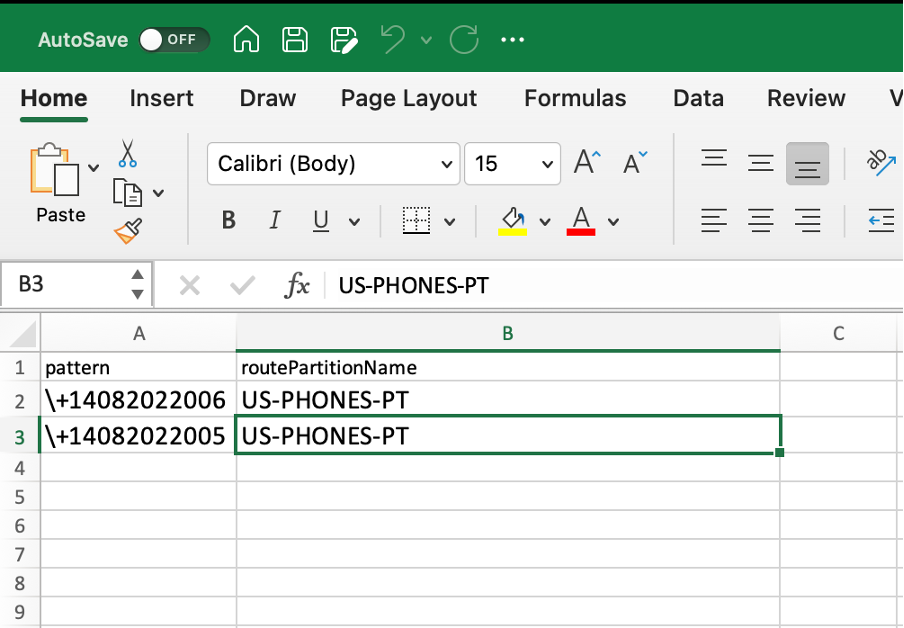
You may pay attention to the variable value and the little format changes in XML request in the send_remove_line_request Python function.
Alternate Method to generate Python code
Additionally, you can utilize the POSTMAN tool. Simply copy the XML code, and POSTMAN will perform the basic conversion from XML format to your desired programming language. Below is a screenshot for reference.
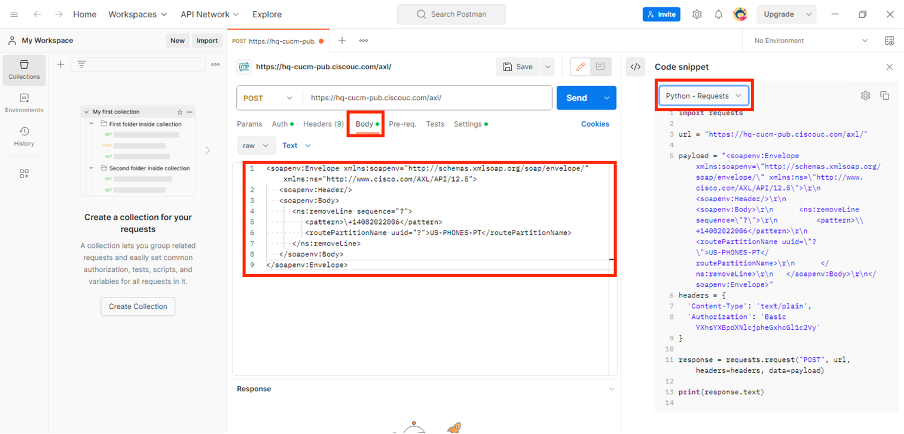
Under the body section, you can copy the raw XML code generated by SOAP UI application and then you can move the right panel where you can choose your desired language, please note that the code generated will be a basic structure and you will have put effort to make it a working one.
great, thanks for sharing the git link